
I have been waiting for this call my entire life-The chance to host Uncle Murph’s birthday celebration. The best part was creating your own individualized version of his favorite cake from a recipe known only by my Aunt Kate and a few chosen family members.
With shopping list in hand, I was off to the market. Arriving back at home with the bounty of ingredients laid out before me measured and in order. However, something was awry, nothing but space where the most vital ingredient was supposed to be. Somehow, I overlooked it. Uncle Murph’s cake would not happen without a return trip to the market. Nevertheless, out of chaos comes opportunity because that day, the idea for a web app was formed.
My web application was not just your run of the mill shopping application, but a fail-safe meal (s) prep guide targeting anyone who returned from the market missing critical items. Applying Python with JavaScript for utility and function, a month of my time and 100+ lines of code later my Chef’s Fail-safe Prep App was complete. (Not so fast!)
A recommended security code review revealed that I would need at a minimum 500+ additional of lines of code to account for all the things that could and most likely would go wrong.
Wait, what? Stressed throughout my education in writing code was that the internet was built for ‘openness and speed,’ not for security. Security could always be added in at a later time. However, the development of my app imparted with me that writing good code is an enormous task. If not done correctly, a range of execution issues and errors can occur including two very well known web exploits; Cross-site request forgery (XSRF) and Cross-site scripting (XSS). Just what are these exploits?
- “XSRF attacks trick a user’s browser to send a forged HTTP request, including the user’s session cookie and the authentication information to a vulnerable web application. The XSRF allows an attacker to force the user’s browsers to generate requests allowing attackers to update account details, make purchases, logout, and login.” Wikipedia
- “XSS attacks occurs when malicious scripts are injected into trusted websites. The malicious script is passed to the end user’s browser. Once executed, the malicious scripts (JavaScript, VBScript, ActiveX, Flash, or HTML) can access any cookies, session tokens, or sensitive information retained by your browser and used with that site. XSS attacks allow for the attacker to gain a high level of control over the user’s system and communicate directly with the site that the user is connected.” Wikipedia
The fundamental difference between XSRF and XSS is inside the ‘victim’s browser.’ An XSRF goal is to exploit the trust that a website has in the visitor’s browser While the XSS is intended to exploit the trust the user has for a specific internet site.
For a deeper understanding of XSRF and XSS and other vulnerabilities can be found in the Open Web Application Security Project (OWASP) Top-10 list; which identifies the most critical application security risks. The top-10 is an industry best practice in preventing web app vulnerabilities and is utilized by many individuals and security organizations.OWASP
The amount of data managed by web apps is growing exponentially, driven by business demand. From online banking to your next Uber ride and everything in between. Web applications are entrusted to manage our most confidential and personal information securely; failing to grasp the nature of this trust can be hazardous. On balance, a single vulnerability can lead to data breaches; it can also result in the theft of Personally identifiable information (PII) which often proves the most costly and detrimental to organizations. Negative headlines, financial and reputational penalties, while legal and regulatory sanctions can quickly escalate into the millions of dollars.
Two worthy mentions of web app attacks include Yahoo! And Ashley Madison.
Yahoo!-
- Multiple data breaches in 2012, 2013, 2014
- Estimated 1 Billion users affected.
- Yahoo! Proprietary Source Code was appropriated.
- PII compromised including:
- Names
- Telephone Numbers
- Dates of Birth
- Encrypted Passwords
- Unencrypted security questions
Ashely Madison-
- 11+ million passwords appropriated.
- 30+ million private accounts compromised.
- Identities exposed and targeted directly.
- Organizations associated with Ashley Madison may face both financial and reputational damages.
In Verizon’s 2016 Data Breach Investigations Report (DBIR), the report identified, web application attacks accounted for a total of 5,334 total incidents, while 908 were responsible for confirmed data disclosure. Moreover, “95 percent of the confirmed web app breaches were financially motivated.”
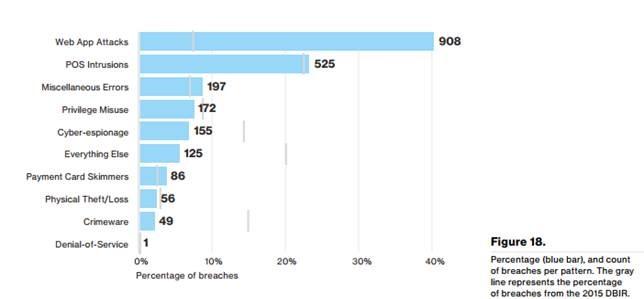
Source: Verizon
When it comes to web app security, complacency is not an option. Unfortunately, Secure coding is often a postscript. Until of course, a security breach is exposed, and then it is too late. Developers need to incorporate best practices into their development. Moreover, it is not satisfactory to find a few vulnerabilities during testing; you need to uncover them all. The risks are inestimable.
Below, find my ultimate shopping list helper written in Python Try it. Have fun! Learn!
shopping_list = []
def remove_item(idx):
index = idx -1
item = shopping_list.pop(index)
print(“Remove {}.”.format(item))
def show_help():
print(“\nSeperate each item with a comma.”)
print(“Type DONE to quit, SHOW to see the current list, and HELP to get this message.”)
def show_list():
count = 1
for item in shopping_list:
print(“{}: {}.”.format(count, item))
count += 1
print(“Give me a list of things to shop for.”)
show_help()
while True:
new_stuff = input(“> “)
if new_stuff == “DONE”:
print(“\nHere is your list:”)
show_list()
break
elif new_stuff == “HELP”:
show_help()
continue
elif new_stuff == “SHOW”:
show_list()
continue
elif new_stuff == “REMOVE”:
show_list()
idx = input(“Which item? Tell me the number. “)
remove_item(int(idx))
continue
else:
new_list = new_stuff.split(“,”)
index = input(“Add this at a certian spot? Press enter for the end of the list. ”
” or give me a number. Currently {} items in the list.”.format(
len(shopping_list)))
if index:
spot = int(index) -1
for item in new_list:
shopping_list.insert(spot, item.strip())
else:
for item in new_list:
shopping_list.append(item.strip())
Cybersecurity is a shared responsibility. Stop. Think. Connect.